Programming a robot might sound complicated, but tools like Python make it surprisingly simple for beginners. Plus, with AI-powered robots, coding isn’t always necessary anymore.
Read on to learn more about:
- A step-by-step guide to programming your first robot
- Key programming concepts for beginners
- Tools and software you need
- Real-world applications of robot programming
- Common challenges and how to overcome them
What does it mean to program a robot?
In simple terms, programming a robot involves writing a set of commands that the robot follows to achieve a goal — whether that's vacuuming your living room or tending a CNC machine in your shop floor.
You could use manual programming, where the robot's movements are manually adjusted. Or, for those just starting, there's block-based coding (think drag-and-drop programming). If you're ready to dive deeper, popular text-based languages like Python and C++ can give you even more granularity and control of your bot’s behavior.
Step-by-step guide to programming a robot
So, you’ve got a robot, and you’re ready to make it do your bidding (it’s not as ominous as it sounds). But how do you actually start programming it? No worries — let’s break it down. Think of this as a quick course in how to program a robot for beginners.
Pick the perfect robot (and environment!)
Before you get into the coding trenches, you’ll need the right bot and place for it to shine.
Here’s how to pick:
- Match your bot to your mission: Are you just starting? Grab a beginner-friendly robot like LEGO Mindstorms or VEX IQ. Are you feeling a bit more adventurous? Dive into Arduino-powered bots or industrial-grade robots like those from Standard Bots.
- Don’t forget the surroundings: Will your robot be working in a factory, your garage, or your living room? The environment plays a big role in what you choose and how you program it.
- Educational, hobby, or hardcore: If it’s for learning or fun, stick with easy-to-use robots. If you’re getting serious (hello, business automation), go with a more advanced model.
Learn a programming language
Choosing the right programming language is crucial for communicating with your robot. Here are some popular languages to consider:
- Python — like chatting with an old friend: Easy to learn and versatile, but for more complex or performance-critical tasks, languages like C++ might be preferred.
- C++ — the coding guru stuff: If you need speed and control, C++ is going to be your top pick. It’s tougher to learn but worth it if you want more power.
- ROS — for robotics pros: The Robot Operating System (ROS) is your tool of choice when you’re getting into advanced robotics. It’s not a language but a framework that makes complex programming easier.
Understand the robot’s insides (and sensors)
You can’t program a robot if you don’t know how its parts work. Think of it like understanding the tools before building the house.
Let’s take a closer look:
- The sensors: From cameras to infrared sensors, these are how your robot "perceives" the world. Get familiar with them because they’ll play a big role in what your robot does.
- Motors powering up the robot’s movement: Your robot’s motors do the heavy lifting. Knowing how they work is key to making sure your bot doesn’t just sit there.
- All connected: Make sure your hardware and software are speaking the same language. Otherwise, your bot’s going to have a hard time following your instructions.
Set up a coding camp
Now that you know what you’re dealing with, it’s time to set up the environment where you can actually do all this — aka your coding lab.
Think of it like this:
- Install the essentials: For simpler robots, you’ll use tools like Arduino IDE or VEXcode. More advanced bots? It's time to set up ROS and get all the right libraries in place.
- Connect the dots: Make sure everything is plugged in and connected properly. A robot with no power or sensors hooked up is just a very expensive paperweight.
- Get comfy with your dev environment: Ensure your workspace is equipped with the necessary tools, from software to power sources, to streamline coding and testing.
The major programming concepts to keep in mind
Now that your robot is set up and moving, it’s time to get into the core programming concepts that will make it more than just fancy circuity.
Loops and conditionals: How your bots learn to think
Loops and conditionals let you tell your robot to do something over and over or make decisions based on input.
- Loops — like a playlist on repeat: Loops allow your robot to do the same action multiple times. Whether it’s making a robot arm lift repeatedly or having your vacuum keep circling that stubborn dirt pile, loops are your go-to.
- Conditionals — if this, then that: If your robot sees an obstacle, it should go around it, right? That’s where conditionals come in. It’s like saying, “Hey, robot, if X happens, then do Y. Otherwise, do Z, okay, my man?” (Well, maybe not like that.)
- Why this matters: Without loops and conditionals, your robot would just follow instructions blindly — no decision-making, no reacting to its surroundings. You want a smart robot, not a robot-zombie.
Functions and modular programming: Robo-multitasking
If you don’t want to write the same code over and over, functions can help you massively streamline this process.
Here’s how:
- One code to rule them all: A function is just a chunk of code that you can reuse whenever you need it. Instead of writing “move forward” 100 times, just put it in a function and call it whenever necessary.
- Modular programming — the building blocks: Breaking your code into smaller, reusable parts (modules) keeps things simple and organized. Plus, it’s easier to troubleshoot when things go wrong.
- The beauty of it all: Imagine being able to tweak one function, and suddenly, your whole program improves. Wouldn’t that be the day? That’s what functions can help you do.
Real-time control: Making adjustments on the fly
Robots don’t work in static environments, so being able to react in real time is like suddenly flipping the channel and watching Braveheart right after a Tommy Wiseau movie.
Here’s why this is so revolutionary:
- It’s like live TV: Your robot needs to adjust instantly based on sensor feedback to avoid obstacles or interact with moving objects. Real-time programming helps it react as things happen.
- Think fast: Your robot’s sensors feed it information constantly, and it has to make decisions on the fly. You’ll need to write code that can handle these quick changes.
- The cool part: Once your robot can make real-time decisions, it’s not just following commands — it’s thinking on its feet (or wheels).
Tools and software for robot programming
You need the right tools to get the job done. Robot programming means using the right platforms, simulators, and libraries to bring your robot to life. (Yes, you can shout, “It’s alive! If you want to, this would be the appropriate time to do it.)
Development environments
Before you even think about testing your code, you’ll need a good development environment to write it in.
Here’s where to start:
- Arduino IDE — simple but sturdy: If you’re working with beginner robots, this is a great choice. It’s easy to use, and you can quickly start coding and uploading instructions to your robot.
- ROS — the robotic mainstay: ROS is a widely used framework for advanced robotics, but it can have a steep learning curve for beginners.
- VEXcode — great for learning: Designed for educational robots like VEX IQ, this is perfect for beginners or classrooms. It keeps things straightforward while teaching the essentials.
Simulators
You don’t always want to test your code on a real robot, especially if there's a chance it might crash into a wall (ouch).
That’s where simulators come in:
- Gazebo — virtual robot heaven: This popular simulator lets you test your robot in virtual environments. Perfect for seeing how your robot would react without risking any hardware (or employees).
- Webots — a sandbox for robots: Another great tool for running your robot through different scenarios in a virtual space, making it easier to debug your code before hitting the real world.
- Why you need them: These simulators let you play around without worrying about wrecking your robot (or your shop floor).
Libraries and frameworks
Why write everything from scratch when other programmers have already done the heavy lifting?
Let’s answer that real quick:
- ROS libraries — plug and play: ROS comes with a bunch of libraries that handle everything from sensor integration to motion planning. Just drop them in, and you’re good to go.
- OpenCV — simplifying robot vision: Want your robot to “see”? OpenCV is a library specifically for computer vision jobs. Perfect for adding cameras and visual recognition to your bot.
- The shortcut you need: Using libraries cuts down your programming time and helps you avoid common mistakes — no need to start from square one!
Applications of robot programming
Programming robots isn't just some high-tech hobby — it's powering industries, education, research, and more.
Let’s take a look at some industries where robots shine:
Industrial automation: Robotic nirvana
When we talk about robots in industries, we’re talking about serious business. Robots can perform the same stuff day in and day out.
Here’s how:
- Robots that never get tired: In factories, robots are often used for welding, painting, or packaging — stuff that needs to happen precisely, over and over again. They’ll do the same task all day without error.
- Saving time and cutting costs: Companies love robots because they reduce human errors, speed up production, and lower costs. For example, a robotic arm programmed to assemble parts on a car production line can work faster than a human, with fewer mistakes.
- Tackling the dirty stuff: Some jobs just aren’t safe or fun for humans. That’s why robots are common in industries like mining or chemical production, where workers can face hazards. They can do the dirty work while keeping people out of harm's way.
Educational robots: Learning with bots
Robots aren’t just for industry; they’re becoming a staple in classrooms, too. Educational robots help kids and beginners learn about programming in a hands-on, engaging way.
See how:
- LEGO Mindstorms — fun and functional: Who knew you could learn programming by playing with LEGOs? LEGO Mindstorms are perfect for beginners, letting you build and program robots without needing any experience. (Plus, let’s face it, Mindstorms is just a cool name.)
- VEX IQ — next-level learning: A bit more advanced than LEGO, VEX IQ robots teach more than just coding. You’ll learn about engineering and robotics while working on your programming skills. Schools use it to get students excited about STEM fields.
- Why it matters: These robots make programming fun and accessible. Instead of staring at code all day, you’re building something that moves, responds, and interacts. It’s a great way to learn by doing, and it’s shaping the next generation of engineers and programmers.
Research and AI integration: Robots on the cutting edge
The research world has a special love for robots, and it’s easy to see why. Robots, combined with AI, are helping push the boundaries of what we can do in science, medicine, and beyond.
This is how superintelligence is helping:
- Robots as research assistants: Need to repeat the same experiment hundreds of times? Robots excel at tasks requiring extreme precision and consistency, handling repetitive work with accuracy that humans might find difficult to replicate.
- AI integration for smarter bots: By combining robotics with artificial intelligence, researchers are creating robots that can make decisions on their own. For example, a robot in a hospital could deliver medication, navigate around patients, and adjust its behavior based on real-time data.
- Into the far reaches: Robots are also essential for research in places humans can’t easily go, like the deep ocean or outer space. NASA’s Mars rovers, for instance, can explore the Red Planet and send back vital data. AI helps them adapt to the terrain and make decisions on the fly.
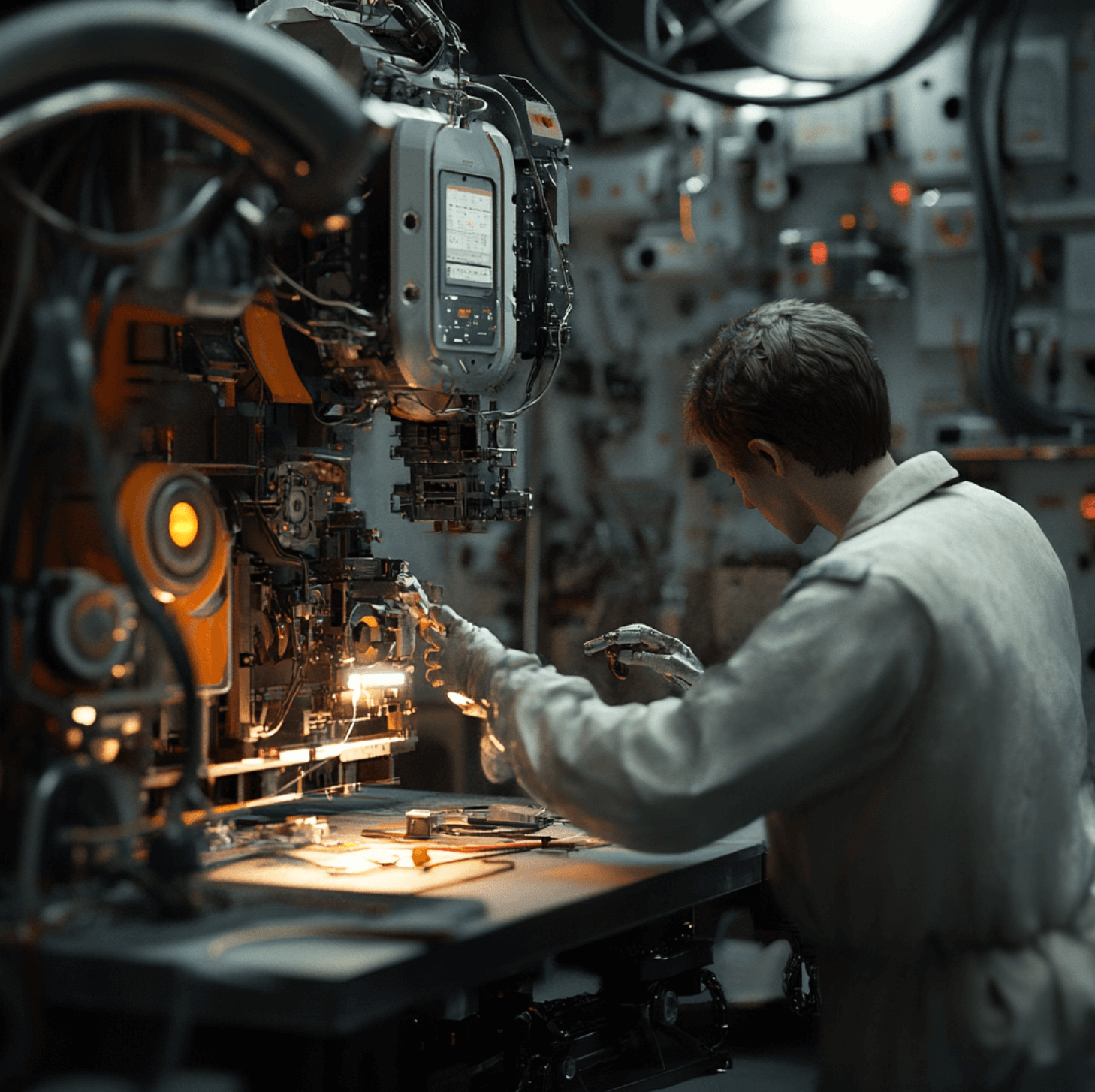
Challenges in robot programming
While programming robots is rewarding, it comes with its share of challenges. From technical hiccups to the time it takes to get everything just right, let’s explore the obstacles you might face.
Let’s take a closer look:
- Hardware integration, or the picky robot syndrome: Getting sensors, motors, and processors to communicate smoothly can be a real puzzle — one wrong move and the whole system goes haywire.
- Debugging, the eternal struggle: Finding and fixing errors isn’t just about the code. Timing, sensor input, and real-world conditions all add layers of complexity (and frustration).
- Development time — it all takes a while: Programming robots is an iterative process. Be ready to test, tweak, and repeat until your bot behaves exactly the way you want.
- Hardware limitations, the hard ceiling: No matter how good your programming is, your robot’s physical components — motors, sensors, batteries — have limits. Don’t expect miracles from a machine that’s built for a more modest fare.
Summing up
Learning how to program a robot can open up a whole world of possibilities. We’re talking about anything from automating repetitive stuff to creating robots that learn and adapt as they go along.
We’ve covered the essentials, from picking the right robot and coding environment to diving into advanced programming concepts and facing the inevitable challenges along the way.
We’re not going to sugarcoat it: The journey of robot programming is all about persistence, creativity, and problem-solving. The payoff of seeing your robot in action is worth every second you spend beating your head against your keyboard.
Want to get a simpler, more streamlined option for your shop floor, though? Read on!
Next steps with Standard Bots’ AI robot
Ready to add a high-tech robot to your team? RO1 by Standard Bots is the six-axis cobot arm that takes the hassle out of robot programming. With minimal setup and a user-friendly interface, you don’t need programming knowledge to get it going.
- The total brainy package: With AI on par with GPT-4 and a no-code interface, RO1 is incredibly easy for anyone to use — zero tech know-how needed.
- Budget-friendly: RO1 won’t break the bank. It’s half the price of comparable models, making automation accessible to everyone.
- Fast & strong: Best-in-class payload capacity of 18kg, and it moves fast. You’ll get more done in less time.
- Safety features: Built-in sensors, machine vision, and collision detection guarantee the safety of your team while RO1 handles the tough jobs.
Ready to see what the RO1 can do for you? Get in touch with our team today and try it risk-free for 30 days. We will assist with setup, ensuring you're ready to implement it seamlessly into your workflow.
Join thousands of creators
receiving our weekly articles.